Hello everybody, I'm trying to port my custom vehicle suspension that I created in Unreal Engine to Godot, this is the algorithm in Unreal:
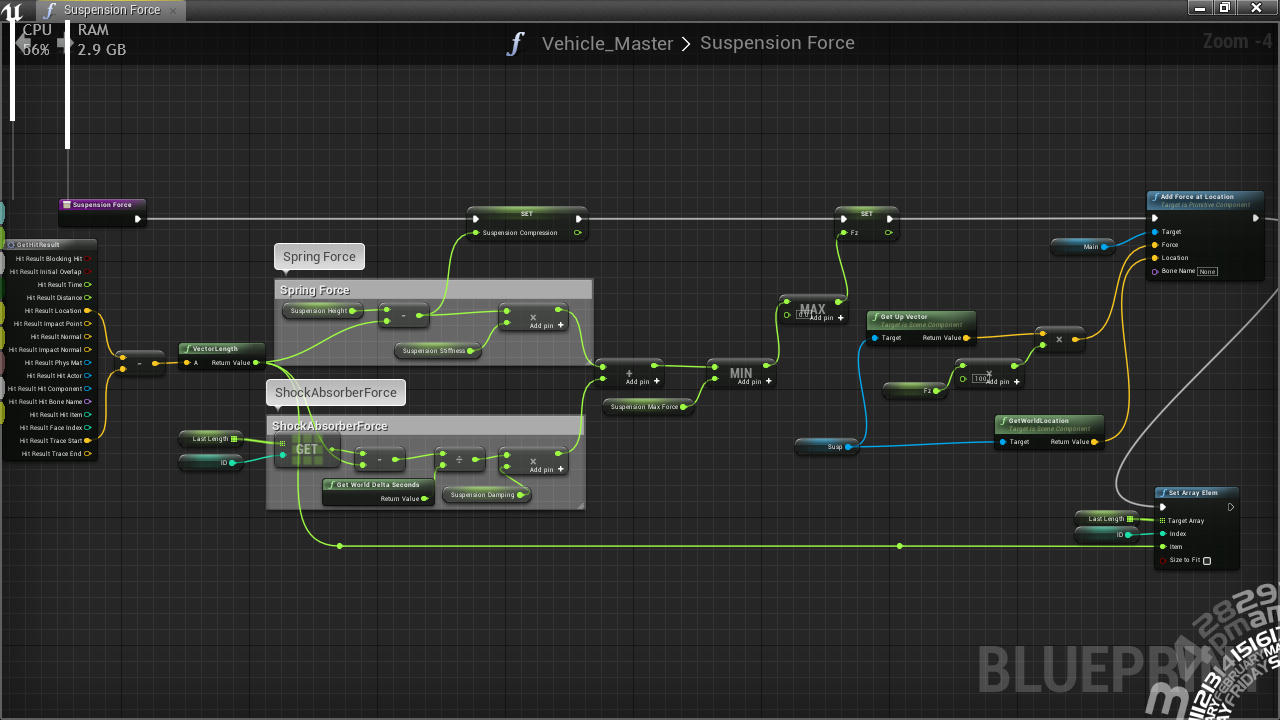
It's pretty simple, and yet it works marvelously, so i tried to replicate it in Godot, with this setup:
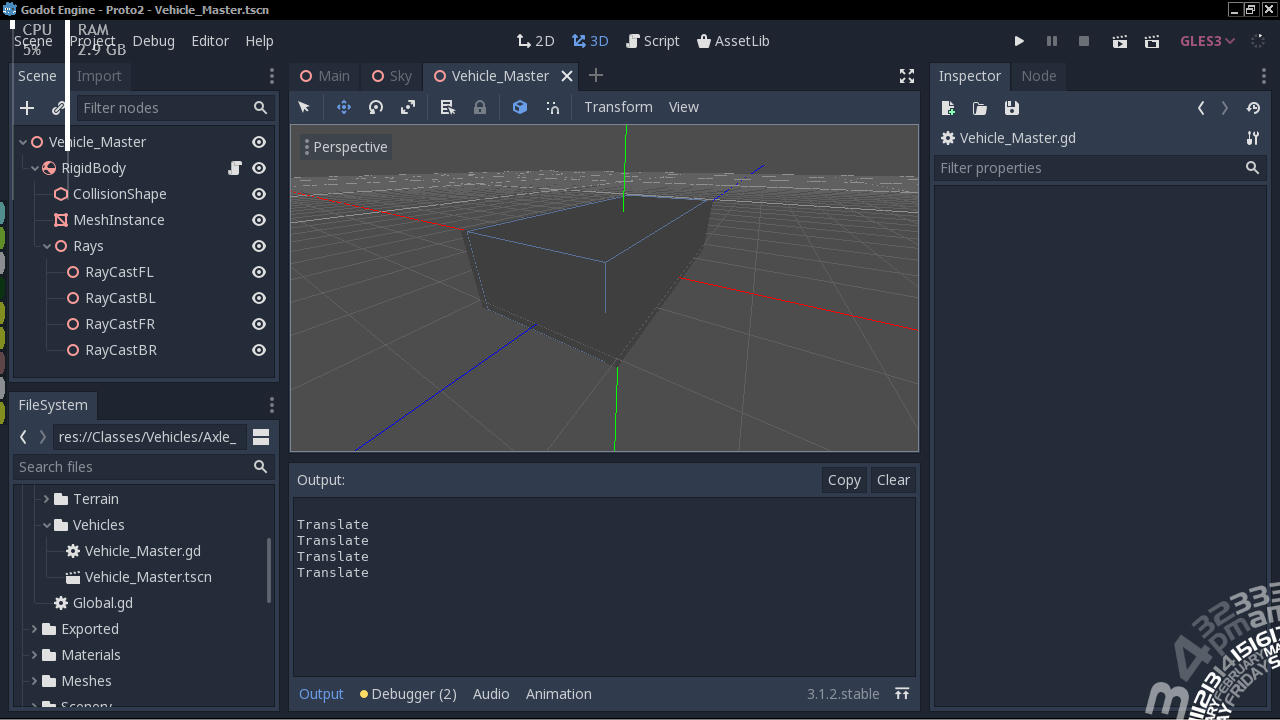
The script is as follows:
extends RigidBody
onready var FR = get_node("Rays/RayCastFR")
onready var FL = get_node("Rays/RayCastFL")
onready var BR = get_node("Rays/RayCastBR")
onready var BL = get_node("Rays/RayCastBL")
export var SuspensionDamping = 4
export var SuspensionStiffness = 4
export var SuspensionMaxForce = 250
export var SuspensionHeight = 0.85
var LastSusLength = [SuspensionHeight,SuspensionHeight,SuspensionHeight,SuspensionHeight]
var gdelta
func _physics_process(delta):
gdelta = delta
func _integrate_forces(state):
# Gravity Force
add_force(Vector3(0.0,-9.8*self.mass,0.0), Vector3(0,0,0))
var space_state = get_world().direct_space_state
for i in range(4):
var R
if i == 0:
R = FR
elif i == 1:
R = FL
elif i == 2:
R = BR
elif i == 3:
R = BL
# Direction to shoot the ray, Suspension Orientation
var dir = R.get_global_transform().basis.y
# Position to shoot the ray, Spring location
var pos = R.get_global_transform().origin
var result = space_state.intersect_ray(pos, pos - dir*0.85)
if result and gdelta != 0.0:
# Suspension Offset
var susoffs = result.position - pos
# Suspension Length
var length = susoffs.length()
# Suspension Compression
var suscomp = SuspensionHeight - length
# Spring Force
var sprforce = suscomp * SuspensionStiffness * self.mass
# Shock Force
var shkforce = ((length - LastSusLength[i]) / gdelta) * SuspensionDamping
# Suspension Force
var Fy = clamp(sprforce + shkforce, -SuspensionMaxForce, SuspensionMaxForce)
add_force(Fy * dir, R.translation)
LastSusLength[i] = length
I had to tweak the values and multiply the force by the vehicle mass, which is 1000
And here is the result:
At first it seems like it wants to work, but then it's almost like the four wheels are not being calculated in the same frame and de-synchronize from one another making the car wobble and go all over the place.
So, is there something i'm missing? Or maybe i need a whole different approach to this?
Can anyone shed some light on the subject?